Registration Statements & Prospectus Filings with Python
The following Python tutorials demonstrate how to access and analyze structured data from registration statements (S-1, S-11, F-1) and prospectuses (424B4) filed with the SEC using the sec-api
Python package and the Registration Statement & Prospectus Data API.
!pip install sec-api
from sec_api import Form_S1_424B4_Api
form_s1_424B4_api = Form_S1_424B4_Api("YOUR_API_KEY")
query = {
"query": "ticker:V",
"from": "0",
"size": "50",
"sort": [{"filedAt": {"order": "desc"}}],
}
response = form_s1_424B4_api.get_data(query)
import json
print(json.dumps(response["data"][0], indent=2))
{
"id": "b4f377b5fcd1c9ea391ba9e8c6c5ab11",
"filedAt": "2008-03-19T17:14:29-04:00",
"accessionNo": "0001193125-08-060989",
"formType": "424B4",
"cik": "1403161",
"ticker": "V",
"entityName": "Visa Inc.",
"filingUrl": "https://www.sec.gov/Archives/edgar/data/1403161/000119312508060989/d424b4.htm",
"tickers": [
{
"ticker": "V",
"type": "Class A Common Stock",
"exchange": "New York Stock Exchange"
}
],
"securities": [
{
"name": "406,000,000 Shares Class A Common Stock"
}
],
"publicOfferingPrice": {
"perShare": 44,
"perShareText": "$44.000",
"total": 17864000000,
"totalText": "$17,864,000,000"
},
"underwritingDiscount": {
"perShare": 1.232,
"perShareText": "$1.232",
"total": 500192000,
"totalText": "$500,192,000"
},
"proceedsBeforeExpenses": {
"perShare": 42.768,
"perShareText": "$42.768",
"total": 17363808000,
"totalText": "$17,363,808,000"
},
"underwriters": [
{
"name": "J.P. Morgan Securities Inc."
},
{
"name": "Goldman, Sachs & Co."
},
{
"name": "UBS Securities LLC"
},
{
"name": "Banc of America Securities LLC"
},
{
"name": "Wachovia Capital Markets, LLC"
},
{
"name": "Citigroup Global Markets Inc."
},
{
"name": "HSBC Securities (USA) Inc."
},
{
"name": "Merrill Lynch, Pierce, Fenner & Smith Incorporated"
},
{
"name": "Piper Jaffray & Co."
},
{
"name": "CIBC World Markets Inc."
},
{
"name": "Mitsubishi UFJ Securities International plc"
},
{
"name": "RBC Capital Markets Corporation"
},
{
"name": "SunTrust Robinson Humphrey Inc."
},
{
"name": "Wells Fargo Securities, LLC"
},
{
"name": "Daiwa Securities America Inc."
},
{
"name": "Mizuho Securities USA Inc."
},
{
"name": "Macquarie Capital (USA) Inc."
},
{
"name": "Credit Suisse Securities (USA) LLC"
},
{
"name": "Santander Investment S.A."
},
{
"name": "TD Securities (USA) LLC"
},
{
"name": "ABN AMRO Incorporated"
},
{
"name": "Barclays Capital Inc."
},
{
"name": "Calyon Securities (USA) Inc."
},
{
"name": "Lehman Brothers Inc."
},
{
"name": "Morgan Keegan & Company, Inc."
},
{
"name": "Scotia Capital (USA) Inc."
},
{
"name": "The Williams Capital Group, L.P."
},
{
"name": "Fox-Pitt Kelton Cochran Caronia Waller (USA) LLC"
},
{
"name": "FirstRand Bank Limited"
},
{
"name": "National Bank of Kuwait"
},
{
"name": "Samsung Securities Co., Ltd."
},
{
"name": "Gardner Rich & Co., Inc."
},
{
"name": "Cowen and Company, LLC"
},
{
"name": "Guzman & Company"
},
{
"name": "Keefe, Bruyette & Woods, Inc."
},
{
"name": "Utendahl Capital Group, LLC"
},
{
"name": "Banco Bilbao Vizcaya Argentaria, S.A."
},
{
"name": "Banco Bradesco BBI S.A."
},
{
"name": "BB&T Capital Markets, a division of Scott & Stringfellow, Inc."
},
{
"name": "BNP Paribas Securities Corp."
},
{
"name": "Loop Capital Markets, LLC"
},
{
"name": "M.R. Beale & Company"
},
{
"name": "Muriel Siebert & Co., Inc."
},
{
"name": "Samuel A. Ramirez & Co., Inc."
},
{
"name": "Dundee Securities Corporation"
}
],
"lawFirms": [
{
"name": "White & Case LLP",
"location": "New York, New York"
},
{
"name": "Davis Polk & Wardwell",
"location": "New York, New York"
}
],
"auditors": [
{
"name": "KPMG LLP"
}
],
"management": [
{
"name": "Joseph W. Saunders",
"age": 62,
"position": "Chairman and Chief Executive Officer"
},
{
"name": "Byron H. Pollitt",
"age": 56,
"position": "Chief Financial Officer"
},
{
"name": "John C. (Hans) Morris",
"age": 49,
"position": "President"
},
{
"name": "William M. Sheedy",
"age": 41,
"position": "Global Head of Corporate Strategy and Business Development"
},
{
"name": "Joshua R. Floum",
"age": 49,
"position": "General Counsel and Corporate Secretary"
},
{
"name": "John M. Partridge",
"age": 58,
"position": "Chief Operating Officer"
},
{
"name": "Ellen Richey",
"age": 58,
"position": "Chief Enterprise Risk Officer"
},
{
"name": "Elizabeth Buse",
"age": 47,
"position": "Global Head of Product"
},
{
"name": "Hani Al-Qadi",
"age": 45,
"position": "Director (Regional director from CEMEA)"
}
],
"employees": {
"total": 5436,
"asOfDate": "2007-12-31",
"perDivision": [],
"perRegion": []
}
}
Loading Large Amounts of Data
Many use cases require loading large amounts of structured data from the Registration Statement & Prospectus Data API, for example loading all data for 424B4 prospectuses from 2010 to 2023. The following code demonstrates how to achieve this by iterating over the range of years from start_year
to end_year
and fetching all 424B4 prospectuses for each month of each year.
The query can be adjusted and tailored to your specific needs. For example, if you are interested in S-1 registration statements, you would simply change the value of the formType
parameter to "S-1"
. Note, the quotation marks around "S-1"
are required otherwise your search query also matches on F-1
and S-11
forms. The updated query_string
would look like this:
f'filedAt:[{start_date} TO {end_date}] AND formType:"S-1"'
In case you are interested in all registration statements and prospectuses, you can simply remove the formType
parameter from the query_string
:
f'filedAt:[{start_date} TO {end_date}]'
def load_data_from_api(start_year=2023, end_year=2024):
years = list(range(start_year, end_year + 1))
months = [f"{month:02d}" for month in range(1, 13)] # 01, 02, ..., 12
data = []
for year in years:
year_counter = 0
for month in months:
has_more = True
query_from = 0
query_size = 50
month_counter = 0
while has_more:
start_date = f"{year}-{month}-01"
end_date = f"{year}-{month}-31"
query_string = (
f'filedAt:[{start_date} TO {end_date}] AND formType:424B4'
)
query = {
"query": query_string,
"from": query_from,
"size": query_size,
"sort": [{"filedAt": {"order": "desc"}}],
}
response = form_s1_424B4_api.get_data(query)
if len(response["data"]) == 0:
break
data += response["data"]
query_from += query_size
month_counter += len(response["data"])
year_counter += month_counter
print(f"✅ {year}-{month} with {month_counter} filings")
print(f"✅ {year} with {year_counter} filings")
return data
forms_424B4 = load_data_from_api(start_year = 2023, end_year = 2023)
✅ 2023-01 with 22 filings
✅ 2023-02 with 39 filings
✅ 2023-03 with 34 filings
✅ 2023-04 with 32 filings
✅ 2023-05 with 33 filings
✅ 2023-06 with 48 filings
✅ 2023-07 with 30 filings
✅ 2023-08 with 36 filings
✅ 2023-09 with 34 filings
✅ 2023-10 with 35 filings
✅ 2023-11 with 40 filings
✅ 2023-12 with 27 filings
✅ 2023 with 410 filings
forms_424B4[:2]
[{'id': '507481881b3e4416d56763903035a635',
'filedAt': '2023-01-31T14:46:26-05:00',
'accessionNo': '0001493152-23-003123',
'formType': '424B4',
'cik': '1879726',
'ticker': 'SIDU',
'entityName': 'Sidus Space Inc.',
'filingUrl': 'https://www.sec.gov/Archives/edgar/data/1879726/000149315223003123/form424b4.htm',
'tickers': [{'ticker': 'SIDU',
'type': 'Class A Common Stock',
'exchange': 'The Nasdaq Capital Market'}],
'securities': [{'name': '2,640,000 shares of Class A Common Stock'},
{'name': 'Pre-Funded Warrants to Purchase 12,360,000 shares of Class A Common Stock'},
{'name': 'Class A common stock'},
{'name': 'Class B common stock'}],
'publicOfferingPrice': {'perShare': 0.3,
'perShareText': '$0.30',
'total': 4500000,
'totalText': '$4,500,000'},
'underwritingDiscount': {'perShare': 0.021,
'perShareText': '$0.021',
'total': 315000,
'totalText': '$315,000'},
'proceedsBeforeExpenses': {'perShare': 0.279,
'perShareText': '$0.279',
'total': 4185000,
'totalText': '$4,185,000'},
'underwriters': [{'name': 'Boustead Securities, LLC'},
{'name': 'EF Hutton, division of Benchmark Investments, LLC'}],
'lawFirms': [{'name': 'Sheppard, Mullin, Richter & Hampton LLP',
'location': 'New York, New York'},
{'name': 'ArentFox Schiff LLP', 'location': 'Washington, DC'}],
'auditors': [{'name': 'BF Borgers CPA PC'}],
'management': [{'name': 'Carol Craig',
'age': 55,
'position': 'Chairwoman and Chief Executive Officer'},
{'name': 'Teresa Burchfield',
'age': 60,
'position': 'Chief Financial Officer'},
{'name': 'Jamie Adams',
'age': 59,
'position': 'Chief Technology Officer and Director'},
{'name': 'Dana Kilborne', 'age': 60, 'position': 'Director'},
{'name': 'Cole Oliver', 'age': 44, 'position': 'Director'},
{'name': 'Miguel Valero', 'age': 59, 'position': 'Director'}],
'employees': {'total': 70,
'asOfDate': '2022-12-30',
'perDivision': [],
'perRegion': []}},
{'id': 'f6bfd35065619cbfda75e7868d4542d5',
'filedAt': '2023-01-30T17:28:57-05:00',
'accessionNo': '0001213900-23-006094',
'formType': '424B4',
'cik': '1939965',
'ticker': 'BREA',
'entityName': 'Brera Holdings PLC',
'filingUrl': 'https://www.sec.gov/Archives/edgar/data/1939965/000121390023006094/ea172411-424b4_brerahold.htm',
'tickers': [{'ticker': 'BREA',
'type': 'Class B Ordinary Shares',
'exchange': 'Nasdaq'}],
'securities': [{'name': '1,500,000 Class B Ordinary Shares'},
{'name': 'Class A Ordinary Shares'}],
'publicOfferingPrice': {'perShare': 5,
'perShareText': '$5.00',
'total': 7500000,
'totalText': '$7,500,000'},
'underwritingDiscount': {'perShare': 0.4,
'perShareText': '$0.40',
'total': 600000,
'totalText': '$600,000'},
'proceedsBeforeExpenses': {'perShare': 4.6,
'perShareText': '$4.60',
'total': 6900000,
'totalText': '$6,900,000'},
'underwriters': [{'name': 'Revere Securities, LLC'}],
'lawFirms': [{'name': 'Bevilacqua PLLC', 'location': 'United States'},
{'name': 'Carmel, Milazzo & Feil LLP', 'location': 'United States'},
{'name': 'Philip Lee LLP', 'location': 'Dublin, Ireland'}],
'auditors': [{'name': 'TAAD LLP'}],
'management': [{'name': 'Sergio Carlo Scalpelli',
'age': 63,
'position': 'Chief Executive Officer and Director'},
{'name': 'Alessandro Aleotti',
'age': 58,
'position': 'Chief Strategy Officer and Director'},
{'name': 'Amedeo Montonati',
'age': 30,
'position': 'Chief Financial Officer'},
{'name': 'Daniel Joseph McClory',
'age': 63,
'position': 'Executive Chairman and Director'},
{'name': 'Alberto Libanori', 'age': 33, 'position': 'Director'},
{'name': 'Christopher Paul Gardner', 'age': 68, 'position': 'Director'},
{'name': 'Pietro Bersani', 'age': 54, 'position': 'Director'},
{'name': 'Goran Pandev', 'age': 39, 'position': 'Director'}],
'employees': {'total': 0,
'asOfDate': '2022-11-30',
'perDivision': [],
'perRegion': []}}]
424B4 Prospectuses by Year
The following example demonstrates how to visualize the number of offering documents (424B4 filings) filed per month per year, from 2000 to 2024. The dataframe df
is created by using the method outlined in the "Loading Large Amounts of Data" section above.
import os
import numpy as np
import pandas as pd
import matplotlib.pyplot as plt
import matplotlib.ticker as mtick
import matplotlib.style as style
params = {
"axes.labelsize": 8, "font.size": 8, "legend.fontsize": 8, "xtick.labelsize": 8,
"ytick.labelsize": 8, "text.usetex": False, "font.family": "sans-serif", "axes.spines.top": False,
"axes.spines.right": False, "grid.color": "grey", "axes.grid": True, "grid.alpha": 0.5,
"grid.linestyle": ":", "axes.grid.axis": "y", "axes.axisbelow": True,
}
plt.rcParams.update(params)
df.head()
filedAt | accessionNo | formType | cik | ticker | entityName | filingUrl | tickers | securities | publicOfferingPrice | underwritingDiscount | proceedsBeforeExpenses | underwriters | lawFirms | auditors | management | employees | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
0 | 2000-01-04 00:00:00-05:00 | 0000950133-00-000008 | S-1/A | 1069502 | VSTY | VARSITYBOOKS COM INC | https://www.sec.gov/Archives/edgar/data/106950... | [{'ticker': 'VSTY', 'type': 'Common Stock', 'e... | [] | {} | {} | {} | [{'name': 'FleetBoston Robertson Stephens Inc.... | [{'name': 'Shaw Pittman', 'location': 'Virgini... | [{'name': 'PricewaterhouseCoopers LLP'}, {'nam... | [{'name': 'Eric J. Kuhn', 'age': 29, 'position... | {'total': 153, 'asOfDate': '1999-12-31', 'perD... |
1 | 2000-01-07 00:00:00-05:00 | 0000950133-00-000038 | S-1/A | 1035096 | WEBM | WEBMETHODS INC | https://www.sec.gov/Archives/edgar/data/103509... | [{'ticker': 'WEBM', 'type': 'Common Stock', 'e... | [{'name': 'shares of common stock'}] | {} | {} | {} | [{'name': 'Morgan Stanley & Co.'}, {'name': 'D... | [{'name': 'Shaw Pittman', 'location': 'Virgini... | [{'name': 'PricewaterhouseCoopers LLP'}] | [{'name': 'Phillip Merrick', 'age': 37, 'posit... | {'total': 150, 'asOfDate': '1999-10-31', 'perD... |
2 | 2000-01-12 00:00:00-05:00 | 0000950133-00-000048 | S-1/A | 1099160 | BBGI | BEASLEY BROADCAST GROUP INC | https://www.sec.gov/Archives/edgar/data/109916... | [{'ticker': 'BBGI', 'type': 'Class A Common St... | [{'name': '6,850,000 Shares Class A Common Sto... | {} | {} | {} | [{'name': 'Credit Suisse First Boston Corporat... | [{'name': 'Latham & Watkins', 'location': 'Was... | [{'name': 'KPMG LLP'}] | [{'name': 'George G. Beasley', 'age': 67, 'pos... | {'total': 565, 'asOfDate': '1999-12-25', 'perD... |
3 | 2000-01-12 00:00:00-05:00 | 0000950131-00-000138 | S-1/A | 1100370 | LNTE | LANTE CORP | https://www.sec.gov/Archives/edgar/data/110037... | [{'ticker': 'LNTE', 'type': 'Common Stock', 'e... | [] | {} | {} | {} | [{'name': 'Credit Suisse First Boston Corporat... | [{'name': 'Katten Muchin Zavis', 'location': '... | [{'name': 'PricewaterhouseCoopers LLP'}] | [{'name': 'Mark Tebbe', 'age': 38, 'position':... | {'total': 344, 'asOfDate': '1999-12-31', 'perD... |
4 | 2000-01-12 00:00:00-05:00 | 0000912057-00-000928 | S-1/A | 1098834 | FRGO | FARGO ELECTRONICS INC | https://www.sec.gov/Archives/edgar/data/109883... | [{'ticker': 'FRGO', 'type': 'Common Stock', 'e... | [{'name': '5,000,000 Shares Common Stock'}] | {} | {} | {} | [{'name': 'Prudential Securities Incorporated'... | [{'name': 'Oppenheimer Wolff & Donnelly LLP', ... | [{'name': 'PricewaterhouseCoopers LLP'}, {'nam... | [{'name': 'Gary R. Holland', 'age': 57, 'posit... | {'total': 165, 'asOfDate': '1999-09-30', 'perD... |
prospectuses = df[df["formType"] == "424B4"].copy()
prospectuses.drop_duplicates(subset="accessionNo", inplace=True)
prospectuses.reset_index(drop=True, inplace=True)
prospectuses["year"] = prospectuses["filedAt"].dt.year
prospectuses["month"] = prospectuses["filedAt"].dt.month
# ensure that every month (1-12) for every year is present
years = prospectuses["year"].unique()
missing_rows = []
for year in years:
for month in range(1, 13):
if len(prospectuses[(prospectuses["year"] == year) & (prospectuses["month"] == month)]) == 0:
missing_rows.append({"year": year, "month": month, "accessionNo": "None"})
missing_rows = pd.DataFrame(missing_rows)
prospectuses = pd.concat([prospectuses, missing_rows], ignore_index=True)
prospectuses.sort_values(by=["year", "month"], inplace=True)
prospectuses_per_month = prospectuses[["year", "month", "accessionNo"]].groupby(
["year", "month"]
).count()
prospectuses_per_month.reset_index(inplace=True)
prospectuses_per_month.rename(columns={"accessionNo": "count"}, inplace=True)
prospectuses_per_month = prospectuses_per_month[
(prospectuses_per_month["year"] >= 2000) & (prospectuses_per_month["year"] <= 2023)
]
prospectuses_per_month
year | month | count | |
---|---|---|---|
0 | 2000 | 1 | 1 |
1 | 2000 | 2 | 5 |
2 | 2000 | 3 | 1 |
3 | 2000 | 4 | 1 |
4 | 2000 | 5 | 2 |
... | ... | ... | ... |
283 | 2023 | 8 | 36 |
284 | 2023 | 9 | 34 |
285 | 2023 | 10 | 36 |
286 | 2023 | 11 | 35 |
287 | 2023 | 12 | 27 |
288 rows × 3 columns
fig, ax = plt.subplots(figsize=(10, 4))
xticks = [
f"{year}-{month}"
for year, month in prospectuses_per_month[["year", "month"]].values
]
ax.plot(
xticks,
prospectuses_per_month["count"],
color="black",
linewidth=1,
)
ax.set_title("424B4 Prospectuses per Month"); ax.set_ylabel("Prospectuses per Month")
ax.set_xticks(xticks); ax.set_xticklabels(xticks, rotation=90, ha="left")
ax.xaxis.set_major_locator(mtick.MultipleLocator(12))
xlabels = [label.get_text() for label in ax.get_xticklabels()]
ax.xaxis.set_major_formatter(mtick.FuncFormatter(lambda x, pos: xlabels[pos][:4]))
ax.grid(axis="y", linestyle=":", alpha=0.5)
ax.spines["top"].set_visible(False); ax.spines["right"].set_visible(False)
# draw vertical lines for each first month of the year, dotted, transparency 0.5,
# with height of the y value for the respective month
for year, month in prospectuses_per_month[["year", "month"]].values:
if month == 1:
ax.vlines(
f"{year}-{month}",
ymin=0,
ymax=prospectuses_per_month[
(prospectuses_per_month["year"] == year)
& (prospectuses_per_month["month"] == month)
]["count"].max(),
linestyle=":",
alpha=0.5,
color="grey",
)
ax.axvspan("2007-1", "2009-1", alpha=0.1, color="red", zorder=-100)
ax.axvspan("2020-1", "2022-1", alpha=0.1, color="red", zorder=-100)
ax.text("2008-1", ax.get_ylim()[1] - 10, "GFC", horizontalalignment="center", verticalalignment="center", color="red", alpha=0.5)
ax.text("2020-12", ax.get_ylim()[1] - 5, "COVID", horizontalalignment="center", verticalalignment="center", color="red",alpha=0.5)
plt.show()
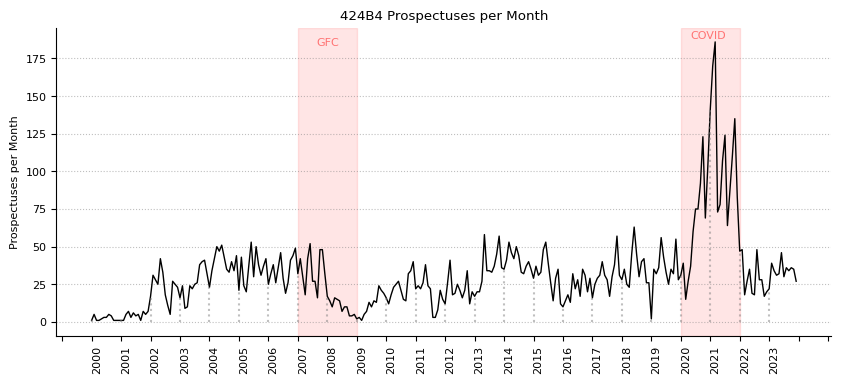
Top 20 Largest Offerings and Offerings by Year
The following example demonstrates how to use the Registration Statements & Prospectuses dataset to find the top 20 largest offerings between 2000 and 2024, as well as the aggregate offering amounts in billions of dollars by year. The dataframe df
contains all extracted data from registration statements (S-1, F-1, S-11) and prospectus filings (424B4) between 2000 and 2024 and was generated using the code provided in the Loading Large Amounts of Data section.
import os
import numpy as np
import pandas as pd
import matplotlib.pyplot as plt
import matplotlib.ticker as mtick
params = {
"axes.labelsize": 8, "font.size": 8, "legend.fontsize": 8, "xtick.labelsize": 8,
"ytick.labelsize": 8, "text.usetex": False, "font.family": "sans-serif", "axes.spines.top": False,
"axes.spines.right": False, "grid.color": "grey", "axes.grid": True, "grid.alpha": 0.5,
"grid.linestyle": ":", "axes.grid.axis": "y", "axes.axisbelow": True,
}
plt.rcParams.update(params)
df.head()
filedAt | accessionNo | formType | cik | ticker | entityName | filingUrl | tickers | securities | publicOfferingPrice | underwritingDiscount | proceedsBeforeExpenses | underwriters | lawFirms | auditors | management | employees | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
0 | 2000-01-04 00:00:00-05:00 | 0000950133-00-000008 | S-1/A | 1069502 | VSTY | VARSITYBOOKS COM INC | https://www.sec.gov/Archives/edgar/data/106950... | [{'ticker': 'VSTY', 'type': 'Common Stock', 'e... | [] | {} | {} | {} | [{'name': 'FleetBoston Robertson Stephens Inc.... | [{'name': 'Shaw Pittman', 'location': 'Virgini... | [{'name': 'PricewaterhouseCoopers LLP'}, {'nam... | [{'name': 'Eric J. Kuhn', 'age': 29, 'position... | {'total': 153, 'asOfDate': '1999-12-31', 'perD... |
1 | 2000-01-07 00:00:00-05:00 | 0000950133-00-000038 | S-1/A | 1035096 | WEBM | WEBMETHODS INC | https://www.sec.gov/Archives/edgar/data/103509... | [{'ticker': 'WEBM', 'type': 'Common Stock', 'e... | [{'name': 'shares of common stock'}] | {} | {} | {} | [{'name': 'Morgan Stanley & Co.'}, {'name': 'D... | [{'name': 'Shaw Pittman', 'location': 'Virgini... | [{'name': 'PricewaterhouseCoopers LLP'}] | [{'name': 'Phillip Merrick', 'age': 37, 'posit... | {'total': 150, 'asOfDate': '1999-10-31', 'perD... |
2 | 2000-01-12 00:00:00-05:00 | 0000950133-00-000048 | S-1/A | 1099160 | BBGI | BEASLEY BROADCAST GROUP INC | https://www.sec.gov/Archives/edgar/data/109916... | [{'ticker': 'BBGI', 'type': 'Class A Common St... | [{'name': '6,850,000 Shares Class A Common Sto... | {} | {} | {} | [{'name': 'Credit Suisse First Boston Corporat... | [{'name': 'Latham & Watkins', 'location': 'Was... | [{'name': 'KPMG LLP'}] | [{'name': 'George G. Beasley', 'age': 67, 'pos... | {'total': 565, 'asOfDate': '1999-12-25', 'perD... |
3 | 2000-01-12 00:00:00-05:00 | 0000950131-00-000138 | S-1/A | 1100370 | LNTE | LANTE CORP | https://www.sec.gov/Archives/edgar/data/110037... | [{'ticker': 'LNTE', 'type': 'Common Stock', 'e... | [] | {} | {} | {} | [{'name': 'Credit Suisse First Boston Corporat... | [{'name': 'Katten Muchin Zavis', 'location': '... | [{'name': 'PricewaterhouseCoopers LLP'}] | [{'name': 'Mark Tebbe', 'age': 38, 'position':... | {'total': 344, 'asOfDate': '1999-12-31', 'perD... |
4 | 2000-01-12 00:00:00-05:00 | 0000912057-00-000928 | S-1/A | 1098834 | FRGO | FARGO ELECTRONICS INC | https://www.sec.gov/Archives/edgar/data/109883... | [{'ticker': 'FRGO', 'type': 'Common Stock', 'e... | [{'name': '5,000,000 Shares Common Stock'}] | {} | {} | {} | [{'name': 'Prudential Securities Incorporated'... | [{'name': 'Oppenheimer Wolff & Donnelly LLP', ... | [{'name': 'PricewaterhouseCoopers LLP'}, {'nam... | [{'name': 'Gary R. Holland', 'age': 57, 'posit... | {'total': 165, 'asOfDate': '1999-09-30', 'perD... |
df_pricing = df[
[
"filedAt",
"accessionNo",
"formType",
"ticker",
"entityName",
"publicOfferingPrice",
"underwritingDiscount",
"proceedsBeforeExpenses",
]
].copy()
df_pricing["publicOfferingPrice.total"] = df_pricing["publicOfferingPrice"].apply(
lambda d: d["total"] if isinstance(d, dict) and "total" in d else None
)
df_pricing["underwritingDiscount.total"] = df_pricing["underwritingDiscount"].apply(
lambda d: d["total"] if isinstance(d, dict) and "total" in d else None
)
ignore_accession_no = [
"0001047469-20-001195",
"0000950137-08-002067",
"0000950137-07-014982",
"0001213900-18-016881",
"0001096350-07-000108",
"0001193125-16-645428",
]
df_pricing = df_pricing[~df_pricing["accessionNo"].isin(ignore_accession_no)]
df_pricing.sort_values(by="publicOfferingPrice.total", ascending=False).head(10)
filedAt | accessionNo | formType | ticker | entityName | publicOfferingPrice | underwritingDiscount | proceedsBeforeExpenses | publicOfferingPrice.total | underwritingDiscount.total | |
---|---|---|---|---|---|---|---|---|---|---|
45027 | 2014-09-15 16:45:24-04:00 | 0001193125-14-341794 | F-1/A | BABA | Alibaba Group Holding Ltd | {'perShare': 68, 'perShareText': 'US$68.00', '... | {'perShare': None, 'perShareText': '', 'total'... | {'perShare': None, 'perShareText': '', 'total'... | 2.503230e+10 | NaN |
45111 | 2014-09-22 06:14:14-04:00 | 0001193125-14-347620 | 424B4 | BABA | Alibaba Group Holding Ltd | {'perShare': 68, 'perShareText': 'US$68.00', '... | {'perShare': 0.8160000000000001, 'perShareText... | {'perShare': 67.184, 'perShareText': 'US$67.18... | 2.176721e+10 | 2.612066e+08 |
66514 | 2020-12-30 17:21:58-05:00 | 0001193125-20-329935 | S-1/A | QS | QuantumScape Corp | {'perShare': 60.5, 'perShareText': '$60.50', '... | {'perShare': 2.15, 'perShareText': '$2.15', 't... | {'perShare': 58.35, 'perShareText': '$58.35', ... | 1.851740e+10 | 6.567710e+05 |
66319 | 2020-12-17 17:30:13-05:00 | 0001193125-20-320220 | S-1 | QS | QuantumScape Corp | {'perShare': 60.5, 'perShareText': '$60.50', '... | {'perShare': 2.5, 'perShareText': '$2.50', 'to... | {'perShare': 58, 'perShareText': '$58.00', 'to... | 1.845940e+10 | 7.624200e+08 |
16062 | 2008-03-19 17:14:29-04:00 | 0001193125-08-060989 | 424B4 | V | Visa Inc. | {'perShare': 44, 'perShareText': '$44.000', 't... | {'perShare': 1.232, 'perShareText': '$1.232', ... | {'perShare': 42.768, 'perShareText': '$42.768'... | 1.786400e+10 | 5.001920e+08 |
34599 | 2012-05-18 16:32:00-04:00 | 0001193125-12-240111 | 424B4 | META | Facebook Inc | {'perShare': 38, 'perShareText': '$38.00', 'to... | {'perShare': 0.418, 'perShareText': '$0.418', ... | {'perShare': 37.582, 'perShareText': '$37.582'... | 1.600688e+10 | 1.760757e+08 |
15963 | 2008-03-11 13:14:31-04:00 | 0000950124-08-001151 | S-1/A | DPH | DELPHI CORP | {'perShare': 38.64, 'perShareText': '$38.64', ... | {'perShare': 1, 'perShareText': '—(1)', 'total... | {'perShare': 59.61, 'perShareText': '$59.61', ... | 1.585257e+10 | 1.000000e+00 |
66448 | 2020-12-23 17:54:52-05:00 | 0001193125-20-326213 | S-1/A | QS | QuantumScape Corp | {'perShare': 60.5, 'perShareText': '$60.50', '... | {'perShare': 1.5, 'perShareText': '$1.50', 'to... | {'perShare': 59, 'perShareText': '$59.00', 'to... | 1.433633e+10 | 3.564461e+08 |
70830 | 2021-06-30 16:36:54-04:00 | 0001193125-21-204763 | 424B4 | XPEV | XPENG INC. | {'perShare': 165, 'perShareText': 'HK$ 165.00'... | {'perShare': 2.228, 'perShareText': 'HK$ 2.228... | {'perShare': 162.772, 'perShareText': 'HK$ 162... | 1.402500e+10 | 1.893375e+08 |
11348 | 2006-11-17 06:02:32-05:00 | 0000950123-06-014259 | S-1/A | SPR | Spirit AeroSystems Holdings, Inc. | {'perShare': 25, 'perShareText': '$25.00', 'to... | {'perShare': None, 'perShareText': '', 'total'... | {'perShare': None, 'perShareText': '', 'total'... | 1.236979e+10 | NaN |
pd.options.display.float_format = "{:,.0f}".format
top_20 = (
df_pricing[df_pricing["formType"] == "424B4"]
.sort_values(by="publicOfferingPrice.total", ascending=False)
.drop_duplicates(subset="ticker")
.head(20)
.sort_values(by="publicOfferingPrice.total", ascending=True)
.reset_index(drop=True)
)
top_20["year"] = top_20["filedAt"].dt.year
years = top_20["filedAt"].dt.year.to_list()
top_20.plot(kind="barh", x="entityName", y="publicOfferingPrice.total", figsize=(6, 4))
ax = plt.gca()
for p, year in zip(ax.patches, years):
ax.annotate(
year,
(p.get_x() + p.get_width(), p.get_y()),
xytext=(0, 0),
textcoords="offset points",
fontsize=7,
)
ax.xaxis.set_major_formatter(mtick.FuncFormatter(lambda x, p: format(int(x) / 1000000000, ",.0f")))
ax.grid(True); ax.set_axisbelow(True); ax.grid(color="gray", linestyle="dashed", alpha=0.3)
ax.spines["top"].set_visible(False); ax.spines["right"].set_visible(False)
ax.yaxis.grid(False), ax.legend().remove()
plt.title("Top 20 Largest Offerings by Total Proceeds\n(2000 - 2024)"); plt.xlabel("Total Proceeds (USD, in billions)")
plt.ylabel(""); plt.tight_layout(); plt.show()
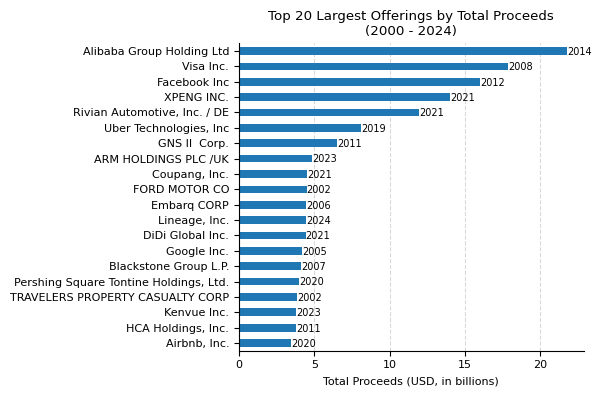
print("Top 20 Largest Offerings by Total Proceeds (2000 - 2024)")
top_20[["entityName", "publicOfferingPrice.total", "year"]].sort_values(
by="publicOfferingPrice.total", ascending=False
).reset_index(drop=True)
Top 20 Largest Offerings by Total Proceeds (2000 - 2024)
entityName | publicOfferingPrice.total | year | |
---|---|---|---|
0 | Alibaba Group Holding Ltd | 21,767,214,800 | 2014 |
1 | Visa Inc. | 17,864,000,000 | 2008 |
2 | Facebook Inc | 16,006,877,370 | 2012 |
3 | XPENG INC. | 14,025,000,000 | 2021 |
4 | Rivian Automotive, Inc. / DE | 11,934,000,000 | 2021 |
5 | Uber Technologies, Inc | 8,100,000,000 | 2019 |
6 | GNS II Corp. | 6,500,000,000 | 2011 |
7 | ARM HOLDINGS PLC /UK | 4,870,500,000 | 2023 |
8 | Coupang, Inc. | 4,550,000,000 | 2021 |
9 | FORD MOTOR CO | 4,500,000,000 | 2002 |
10 | Embarq CORP | 4,484,726,050 | 2006 |
11 | Lineage, Inc. | 4,436,799,978 | 2024 |
12 | DiDi Global Inc. | 4,435,200,000 | 2021 |
13 | Google Inc. | 4,176,983,175 | 2005 |
14 | Blackstone Group L.P. | 4,133,333,354 | 2007 |
15 | Pershing Square Tontine Holdings, Ltd. | 4,000,000,000 | 2020 |
16 | TRAVELERS PROPERTY CASUALTY CORP | 3,885,000,000 | 2002 |
17 | Kenvue Inc. | 3,801,876,320 | 2023 |
18 | HCA Holdings, Inc. | 3,786,000,000 | 2011 |
19 | Airbnb, Inc. | 3,490,000,108 | 2020 |
total = (
df_pricing[df_pricing["formType"] == "424B4"]
.drop_duplicates(subset="ticker")[
["filedAt", "publicOfferingPrice.total", "accessionNo"]
]
.copy()
)
total.drop(columns=["accessionNo"], inplace=True)
total["year"] = total["filedAt"].dt.year
total.drop(columns=["filedAt"], inplace=True)
total.fillna(0, inplace=True)
total = total.apply(pd.to_numeric, errors="coerce").fillna(0)
total
publicOfferingPrice.total | year | |
---|---|---|
8 | 0 | 2000 |
27 | 143,500,000 | 2000 |
29 | 80,000,000 | 2000 |
31 | 75,000,000 | 2000 |
35 | 40,750,000 | 2000 |
... | ... | ... |
84869 | 1,200,000 | 2024 |
84922 | 6,400,000 | 2024 |
84926 | 250,000,000 | 2024 |
84930 | 300,000,000 | 2024 |
84989 | 350,000,000 | 2024 |
5979 rows × 2 columns
total_by_year = total.groupby("year").sum()
total_by_year.plot(kind="bar", figsize=(4, 3))
plt.title("Annual 424B4 Offering Amount per Year\n(2000 - 2024)")
plt.ylabel("Offering Amount\n(USD, in billions)"); plt.xlabel("")
ax = plt.gca()
ax.yaxis.set_major_formatter(mtick.FuncFormatter(lambda x, p: format(int(x) / 1000000000, ",.0f")))
ax.get_legend().remove(), ax.grid(), ax.set_axisbelow(True)
ax.grid(True); ax.set_axisbelow(True); ax.grid(color="gray", linestyle="dashed", alpha=0.3)
ax.spines["top"].set_visible(False); ax.spines["right"].set_visible(False); ax.xaxis.grid(False)
plt.setp(ax.get_xticklabels(), fontsize=7); plt.tight_layout(); plt.show()
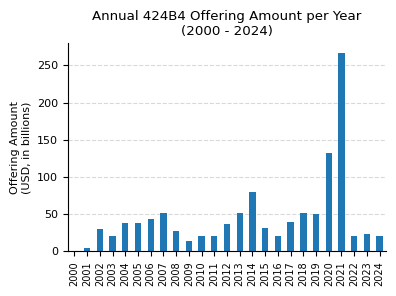
Most Used Law Firms During Securities Offerings (424B4)
The following example shows how to determine the top 20 most often used law firms during securities offerings from 2000 to 2024. As with previous examples, the dataframe df
contains all extracted data from registration statements (S-1, F-1, S-11) and prospectus filings (424B4) between 2000 and 2024 and was generated using the code provided in the Loading Large Amounts of Data section.
import os
import numpy as np
import pandas as pd
import matplotlib.pyplot as plt
import matplotlib.ticker as mtick
params = {
"axes.labelsize": 8, "font.size": 8, "legend.fontsize": 8, "xtick.labelsize": 8,
"ytick.labelsize": 8, "text.usetex": False, "font.family": "sans-serif", "axes.spines.top": False,
"axes.spines.right": False, "grid.color": "grey", "axes.grid": True, "grid.alpha": 0.5,
"grid.linestyle": ":", "axes.grid.axis": "y", "axes.axisbelow": True,
}
plt.rcParams.update(params)
df.head()
filedAt | accessionNo | formType | cik | ticker | entityName | filingUrl | tickers | securities | publicOfferingPrice | underwritingDiscount | proceedsBeforeExpenses | underwriters | lawFirms | auditors | management | employees | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
0 | 2000-01-04 00:00:00-05:00 | 0000950133-00-000008 | S-1/A | 1069502 | VSTY | VARSITYBOOKS COM INC | https://www.sec.gov/Archives/edgar/data/106950... | [{'ticker': 'VSTY', 'type': 'Common Stock', 'e... | [] | {} | {} | {} | [{'name': 'FleetBoston Robertson Stephens Inc.... | [{'name': 'Shaw Pittman', 'location': 'Virgini... | [{'name': 'PricewaterhouseCoopers LLP'}, {'nam... | [{'name': 'Eric J. Kuhn', 'age': 29, 'position... | {'total': 153, 'asOfDate': '1999-12-31', 'perD... |
1 | 2000-01-07 00:00:00-05:00 | 0000950133-00-000038 | S-1/A | 1035096 | WEBM | WEBMETHODS INC | https://www.sec.gov/Archives/edgar/data/103509... | [{'ticker': 'WEBM', 'type': 'Common Stock', 'e... | [{'name': 'shares of common stock'}] | {} | {} | {} | [{'name': 'Morgan Stanley & Co.'}, {'name': 'D... | [{'name': 'Shaw Pittman', 'location': 'Virgini... | [{'name': 'PricewaterhouseCoopers LLP'}] | [{'name': 'Phillip Merrick', 'age': 37, 'posit... | {'total': 150, 'asOfDate': '1999-10-31', 'perD... |
2 | 2000-01-12 00:00:00-05:00 | 0000950133-00-000048 | S-1/A | 1099160 | BBGI | BEASLEY BROADCAST GROUP INC | https://www.sec.gov/Archives/edgar/data/109916... | [{'ticker': 'BBGI', 'type': 'Class A Common St... | [{'name': '6,850,000 Shares Class A Common Sto... | {} | {} | {} | [{'name': 'Credit Suisse First Boston Corporat... | [{'name': 'Latham & Watkins', 'location': 'Was... | [{'name': 'KPMG LLP'}] | [{'name': 'George G. Beasley', 'age': 67, 'pos... | {'total': 565, 'asOfDate': '1999-12-25', 'perD... |
3 | 2000-01-12 00:00:00-05:00 | 0000950131-00-000138 | S-1/A | 1100370 | LNTE | LANTE CORP | https://www.sec.gov/Archives/edgar/data/110037... | [{'ticker': 'LNTE', 'type': 'Common Stock', 'e... | [] | {} | {} | {} | [{'name': 'Credit Suisse First Boston Corporat... | [{'name': 'Katten Muchin Zavis', 'location': '... | [{'name': 'PricewaterhouseCoopers LLP'}] | [{'name': 'Mark Tebbe', 'age': 38, 'position':... | {'total': 344, 'asOfDate': '1999-12-31', 'perD... |
4 | 2000-01-12 00:00:00-05:00 | 0000912057-00-000928 | S-1/A | 1098834 | FRGO | FARGO ELECTRONICS INC | https://www.sec.gov/Archives/edgar/data/109883... | [{'ticker': 'FRGO', 'type': 'Common Stock', 'e... | [{'name': '5,000,000 Shares Common Stock'}] | {} | {} | {} | [{'name': 'Prudential Securities Incorporated'... | [{'name': 'Oppenheimer Wolff & Donnelly LLP', ... | [{'name': 'PricewaterhouseCoopers LLP'}, {'nam... | [{'name': 'Gary R. Holland', 'age': 57, 'posit... | {'total': 165, 'asOfDate': '1999-09-30', 'perD... |
df_lawfirms = df[df['formType'] == '424B4'][["filedAt", "accessionNo", "lawFirms"]].copy()
df_lawfirms = df_lawfirms.explode("lawFirms")
df_lawfirms["lawFirmName"] = df_lawfirms["lawFirms"].apply(
lambda d: d["name"].upper()
if isinstance(d, dict) and "name" in d and d["name"] is not None
else None
)
df_lawfirms["lawFirmLocation"] = df_lawfirms["lawFirms"].apply(lambda d: d["location"] if isinstance(d, dict) and "location" in d else None)
df_lawfirms.drop_duplicates(subset=["accessionNo", "lawFirmName"], inplace=True)
df_lawfirms.head()
filedAt | accessionNo | lawFirms | lawFirmName | lawFirmLocation | |
---|---|---|---|---|---|
8 | 2000-01-25 00:00:00-05:00 | 0000950152-00-000338 | {'name': 'Strauss & Troy, a Legal Professional... | STRAUSS & TROY, A LEGAL PROFESSIONAL ASSOCIATION | Ohio, USA |
8 | 2000-01-25 00:00:00-05:00 | 0000950152-00-000338 | {'name': 'Shearman & Sterling', 'location': 'N... | SHEARMAN & STERLING | New York, USA |
27 | 2000-02-11 00:00:00-05:00 | 0000950133-00-000426 | {'name': 'Shaw Pittman', 'location': 'Virginia... | SHAW PITTMAN | Virginia, USA |
27 | 2000-02-11 00:00:00-05:00 | 0000950133-00-000426 | {'name': 'Davis Polk & Wardwell', 'location': ... | DAVIS POLK & WARDWELL | New York, USA |
29 | 2000-02-11 00:00:00-05:00 | 0000950131-00-001047 | {'name': 'Katten Muchin Zavis', 'location': 'I... | KATTEN MUCHIN ZAVIS | Illinois, USA |
import ast
with open("../output/law-firms-grouped.txt", "r") as f:
law_firms_grouped = ast.literal_eval(f.read())
print([law_firms for law_firms in law_firms_grouped if len(law_firms) >= 4][0])
['ALLEN & GLEDHILL LLP', 'ALLEN & OVERY', 'ALLEN & OVERY LEGAL (VIETNAM) LLC', 'ALLEN & OVERY LLP', 'ALLEN & OVERY S.C.S.']
def get_law_firm_group(law_firm_name):
if law_firm_name is None:
return None
if isinstance(law_firm_name, str) and law_firm_name.strip() == "":
return None
for group in law_firms_grouped:
if law_firm_name in group:
return group[0]
return law_firm_name
df_lawfirms["lawFirmGroup"] = df_lawfirms["lawFirmName"].apply(lambda d: get_law_firm_group(d))
df_lawfirms.head()
filedAt | accessionNo | lawFirms | lawFirmName | lawFirmLocation | lawFirmGroup | |
---|---|---|---|---|---|---|
8 | 2000-01-25 00:00:00-05:00 | 0000950152-00-000338 | {'name': 'Strauss & Troy, a Legal Professional... | STRAUSS & TROY, A LEGAL PROFESSIONAL ASSOCIATION | Ohio, USA | STRAUSS & TROY, A LEGAL PROFESSIONAL ASSOCIATION |
8 | 2000-01-25 00:00:00-05:00 | 0000950152-00-000338 | {'name': 'Shearman & Sterling', 'location': 'N... | SHEARMAN & STERLING | New York, USA | SHEARMAN & STERLING |
27 | 2000-02-11 00:00:00-05:00 | 0000950133-00-000426 | {'name': 'Shaw Pittman', 'location': 'Virginia... | SHAW PITTMAN | Virginia, USA | SHAW PITTMAN |
27 | 2000-02-11 00:00:00-05:00 | 0000950133-00-000426 | {'name': 'Davis Polk & Wardwell', 'location': ... | DAVIS POLK & WARDWELL | New York, USA | DAVIS POLK & WARDWELL |
29 | 2000-02-11 00:00:00-05:00 | 0000950131-00-001047 | {'name': 'Katten Muchin Zavis', 'location': 'I... | KATTEN MUCHIN ZAVIS | Illinois, USA | KATTEN MUCHIN ZAVIS |
df_lawfirms_pivot = df_lawfirms.pivot_table(
index="lawFirmGroup",
columns=df_lawfirms["filedAt"].dt.year,
values="accessionNo",
aggfunc="count",
fill_value=0,
)
df_lawfirms_pivot.sort_index(inplace=True)
df_lawfirms_pivot = df_lawfirms_pivot[df_lawfirms_pivot.sum(axis=1) >= 10]
df_lawfirms_pivot
filedAt | 2000 | 2001 | 2002 | 2003 | 2004 | 2005 | 2006 | 2007 | 2008 | 2009 | ... | 2015 | 2016 | 2017 | 2018 | 2019 | 2020 | 2021 | 2022 | 2023 | 2024 |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
lawFirmGroup | |||||||||||||||||||||
AKERMAN LLP | 0 | 0 | 0 | 0 | 0 | 2 | 2 | 3 | 0 | 0 | ... | 1 | 2 | 2 | 0 | 0 | 0 | 2 | 1 | 2 | 1 |
AKIN GUMP LLP | 0 | 0 | 1 | 3 | 1 | 3 | 3 | 9 | 3 | 1 | ... | 1 | 1 | 2 | 1 | 3 | 5 | 3 | 0 | 0 | 1 |
ALLBRIGHT LAW | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | ... | 4 | 0 | 0 | 2 | 3 | 2 | 8 | 2 | 5 | 4 |
ALLEN & GLEDHILL LLP | 0 | 0 | 0 | 8 | 7 | 3 | 0 | 0 | 0 | 1 | ... | 1 | 0 | 2 | 1 | 1 | 0 | 1 | 0 | 0 | 1 |
ALLENS ARTHUR ROBINSON | 0 | 0 | 2 | 6 | 16 | 3 | 0 | 0 | 0 | 0 | ... | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 |
... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... |
WINSTON & STRAWN LLP | 0 | 0 | 0 | 0 | 4 | 4 | 2 | 1 | 0 | 1 | ... | 1 | 5 | 5 | 9 | 9 | 18 | 25 | 5 | 11 | 3 |
WYRICK ROBBINS YATES & PONTON LLP | 0 | 0 | 1 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | ... | 4 | 3 | 1 | 3 | 2 | 1 | 1 | 0 | 1 | 1 |
YIGAL ARNON & CO. | 0 | 0 | 0 | 0 | 0 | 0 | 1 | 2 | 0 | 0 | ... | 2 | 0 | 0 | 0 | 0 | 1 | 1 | 0 | 0 | 0 |
ZHONG LUN LAW FIRM | 0 | 0 | 0 | 0 | 0 | 1 | 0 | 1 | 0 | 1 | ... | 1 | 1 | 3 | 5 | 5 | 4 | 3 | 4 | 4 | 4 |
ZYSMAN, AHARONI, GAYER AND SULLIVAN & WORCESTER LLP | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | ... | 1 | 7 | 3 | 6 | 6 | 6 | 0 | 0 | 0 | 0 |
219 rows × 25 columns
df_lawfirms_pivot.sum(axis=1).sort_values(ascending=False)[:10].sort_values(
ascending=True
).plot(kind="barh", figsize=(4, 2.5))
plt.title("Top 10 Most Used Law Firms for 424B4 Prospectuses\n(2000 - 2024)")
plt.xlabel("# Prospectuses"), plt.ylabel("")
ax = plt.gca()
ax.grid(color="gray", linestyle="dashed", alpha=0.3); ax.set_axisbelow(True); ax.yaxis.grid(False)
ax.spines["top"].set_visible(False), ax.spines["right"].set_visible(False)
ax.xaxis.set_major_formatter(mtick.FuncFormatter(lambda x, p: format(int(x), ",")))
plt.show()
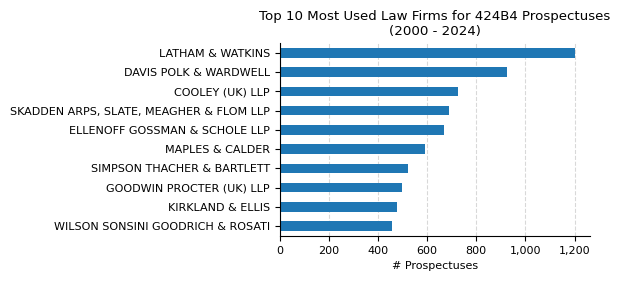
print("Top 10 Most Used Law Firms for 424B4 Prospectuses (2000 - 2024)")
df_lawfirms_pivot.sum(axis=1) \
.sort_values(ascending=False)[:10] \
.to_frame() \
.reset_index() \
.rename(columns={0: "# Prospectuses", "lawFirmGroup": "Law Firm"})
Top 10 Most Used Law Firms for 424B4 Prospectuses (2000 - 2024)
Law Firm | # Prospectuses | |
---|---|---|
0 | LATHAM & WATKINS | 1201 |
1 | DAVIS POLK & WARDWELL | 927 |
2 | COOLEY (UK) LLP | 726 |
3 | SKADDEN ARPS, SLATE, MEAGHER & FLOM LLP | 690 |
4 | ELLENOFF GOSSMAN & SCHOLE LLP | 670 |
5 | MAPLES & CALDER | 591 |
6 | SIMPSON THACHER & BARTLETT | 522 |
7 | GOODWIN PROCTER (UK) LLP | 496 |
8 | KIRKLAND & ELLIS | 479 |
9 | WILSON SONSINI GOODRICH & ROSATI | 458 |